[Python] 메타의 대규모 AI 언어모델 Llama3 설치 및 사용방법 [01]
메타에서 AI기술의 발전을 가속화 할 수 있도록 대규모 언어 모델(LLM)을 오픈소스로 공개했습니다.OpenAI의 GPT로 테스트를 진행하려면 카드를 등록해서 API비용도 지불해야되고 여러 가지 껄끄러
dev-drive.tistory.com
라마3 모델의 한국어 처리 능력을 향상시키려면 한국어로 파인튜닝된 모델을 사용하거나,
LangChain을 통해 한국어로 번역한 후 답변해 달라고 요청하는 등의 방법을 사용할 수 있습니다.
파인튜닝(Fine-tuning)이란?
이미 학습된 머신러닝 모델을 새로운 데이터나 특정 작업에 맞게 추가로 학습시키는 것을 의미합니다.
랭체인(LangChain)이란?
지시사항이나 답변 예시 등 다양한 데이터를 체인으로 연결하여 자연어 처리(NLP)나 검색-증강 생성(RAG)
애플리케이션을 구축하는 데 도움을 주는 프레임워크입니다.
검색-증강 생성(RAG, Retrieval-Augmented Generation)이란?
언어 모델이 답변을 생성하기 전에 관련 정보를 검색하여 신뢰성 있는 답변을 제공할 수 있도록 하는 기술입니다.
언어 모델은 특정 시점을 기준으로 업데이트가 되기 때문에 그 이후에 일어난 일들에 대해서는
학습되지 않아서 그럴듯한 답변을 지어내는 등의 환각 답변을 생성하는 문제가 존재하는데,
이런 경우 관련된 문서를 찾거나 API를 통해서 정확한 정보를 얻은 후 답변을 생성하는 등의 기술을 의미합니다.
🔷 랭체인으로 ollama 모델을 실행하여 간단한 질문을 해보겠습니다.
# 실행하는 데 필요한 라이브러리가 설치되어 있지 않아면 먼저 설치를 진행해주세요 ex) pip install langchain
from langchain_core.prompts import ChatPromptTemplate
from langchain_core.output_parsers import StrOutputParser
from langchain_community.chat_models import ChatOllama
llm = ChatOllama(model="llama3.1:latest")
prompt = ChatPromptTemplate.from_messages([
("system", "You are a helpful AI assistant. Please answer the user's questions kindly. Answer me in Korean no matter what."),
("user", "{input}")
])
chain = prompt | llm | StrOutputParser()
print(chain.invoke({"input": "LLM이 뭐야?"}))
system에 답변을 한국어로 친절하게 해달라고 요청하고 유저의 질문을 입력받는 예제입니다.
이런 식으로 AI에게 역할을 부여해주고 정해진 규칙에 따라 답변을 하도록 지시하는 것을
프롬프트 엔지니어링이라고 합니다.
여기서 prompt, llm, StrOutputParser()을 하나로 연결시키는 것을 체인이라고 표현하며,
StrOutputParser()을 추가한 것은 답변을 문자열로 변환하기 위함입니다

모델에서 모든 답변 생성을 마친 후 한꺼번에 출력되는 모습입니다.
🔷 다음은 챗GPT처럼 한글자씩 바로바로 출력되도록 구현해보겠습니다.
from langchain_core.prompts import ChatPromptTemplate
from langchain_core.output_parsers import StrOutputParser
from langchain_community.chat_models import ChatOllama
llm = ChatOllama(model="llama3.1:latest")
prompt = ChatPromptTemplate.from_messages([
("system", "You are a helpful AI assistant. Please answer the user's questions kindly. Answer me in Korean no matter what."),
("user", "{input}")
])
chain = prompt | llm | StrOutputParser()
for token in chain.stream(
{"input": "LLM이 뭐야?"}
):
print(token, end="")

결과를 보면 한글자씩 바로바로 출력되는 모습을 확인할 수 있으며, 이런 것을 스트리밍 출력이라고 표현합니다.
🦜️🏓 LangServe
랭서브는 모델을 REST API로 배포하여 서비스할 수 있도록 도움을 주는 프레임워크입니다.
🔷 LangServe playground로 테스트하는 예제 구현해보겠습니다.
FastAPI를 통해 api서버를 만들고 랭서브를 사용하기 위한 환경설정을 진행합니다.
pip install -U langchain-cli
langchain app new myApp
이렇게 입력하면 /myApp폴더에 랭서브 구동에 필요한 기본 환경파일들이 생성됩니다.
chain을 따로 파이썬 파일로 만들어 줍니다. (ex. chain.py)
from langchain_core.prompts import ChatPromptTemplate
from langchain_core.output_parsers import StrOutputParser
from langchain_community.chat_models import ChatOllama
llm = ChatOllama(model="llama3.1:latest")
prompt = ChatPromptTemplate.from_messages([
("system", "You are a helpful AI assistant. Please answer the user's questions kindly. Answer me in Korean no matter what."),
("user", "{input}")
])
chain = prompt | llm | StrOutputParser()
/app/server.py에 코드를 입력하여 playground로 간단하게 웹에서 테스트 해볼 수 있습니다.
from fastapi import FastAPI
from fastapi.responses import RedirectResponse
from langserve import add_routes
from chain import chain as myChain
app = FastAPI()
add_routes(
app,
myChain,
path="/ollama"
)
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="localhost", port=8000)
server.py 실행 후 http://localhost:8000/ollama/playground/ 접속
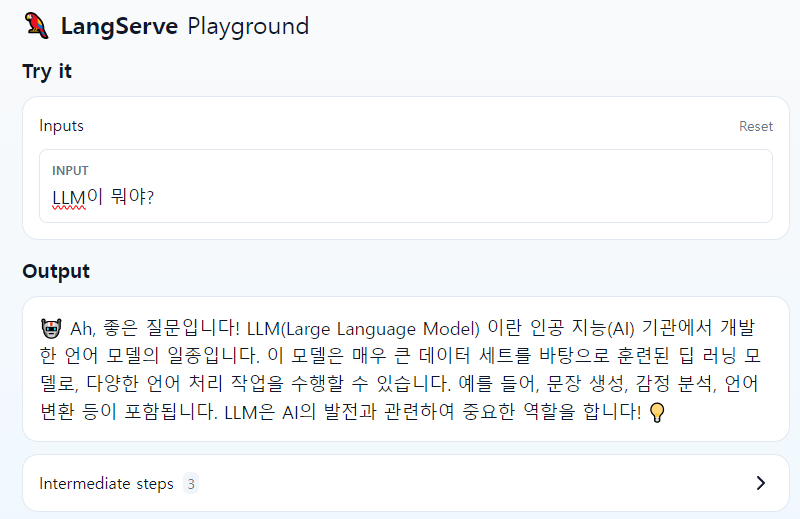
🔷 다음은 채팅 형식으로 테스트하는 예제입니다.
채팅 형식으로 대화하려면 MessagePlaceholder가 필요합니다.
chain.py를 수정해줍니다.
from langchain_core.prompts import ChatPromptTemplate, MessagesPlaceholder
from langchain_core.output_parsers import StrOutputParser
from langchain_community.chat_models import ChatOllama
llm = ChatOllama(model="llama3.1:latest")
prompt = ChatPromptTemplate.from_messages([
("system", "You are a helpful AI assistant. Please answer the user's questions kindly. Answer me in Korean no matter what."),
MessagesPlaceholder(variable_name='messsages1'),
])
chain = prompt | llm | StrOutputParser()
다음은 server.py를 수정해줍니다.
from fastapi import FastAPI
from langserve import add_routes
from chain import chain as myChain
from typing import List, Union
from langserve.pydantic_v1 import BaseModel, Field
from langchain_core.messages import HumanMessage, AIMessage, SystemMessage
class InputChat(BaseModel):
messsages1: List[Union[HumanMessage, AIMessage, SystemMessage]] = Field(
...,
description="The chat messages representing the current conversation.",
)
app = FastAPI()
add_routes(
app,
myChain.with_types(input_type=InputChat),
path="/ollama",
enable_feedback_endpoint=True,
enable_public_trace_link_endpoint=True,
playground_type="chat",
)
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="localhost", port=8000)
동일하게 server.py 실행 후 http://localhost:8000/ollama/playground/ 접속하면 채팅 형식으로 대화할 수 있습니다.
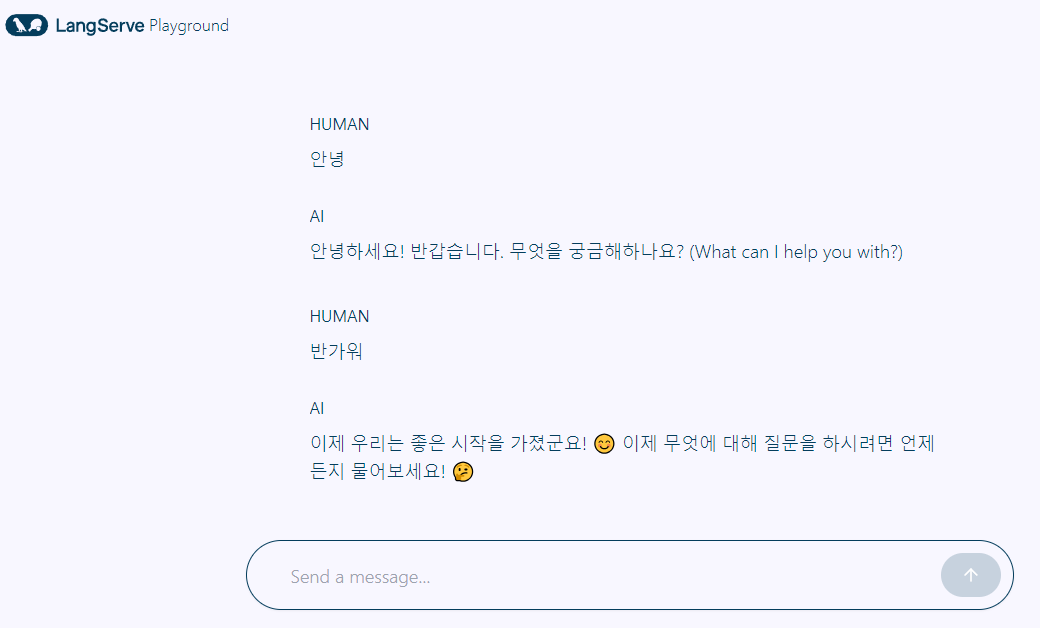
이후에 간단하게 페이지 개발을 하려면 Streamlit 프레임워크를 사용하면 되고,
외부에서도 접속할 수 있게 배포하려면 Ngrok을 사용하면 되니
필요하신분들은 검색해서 구현해보시길 바랍니다😀
다음은 GPU 환경 설정에 대해 알아보겠습니다.
[Python] GPU로 모델을 구동하기 위한 PyTorch + CUDA + cuDNN 설정 방법 [03]
[Python] LangChain으로 Llama3 언어 모델 구동해보기 [02][Python] 메타의 대규모 AI 언어모델 Llama3 설치 및 사용방법 [01]메타에서 AI기술의 발전을 가속화 할 수 있도록 대규모 언어 모델(LLM)을 오픈소스로
dev-drive.tistory.com
'파이썬' 카테고리의 다른 글
[Python] HuggingFace로 오픈소스 AI모델 구동해보기 [04] (1) | 2024.09.10 |
---|---|
[Python] GPU로 AI 모델을 구동하기 위한 PyTorch + CUDA + cuDNN 설정 방법 [03] (0) | 2024.07.29 |
[Python] 메타의 대규모 AI 언어모델 Llama3.1 설치 및 사용방법 [01] (0) | 2024.06.27 |
댓글